OAuth requires the authenticating user to interact with Zoho CRM using the browser. Simply log into your instance of Zoho CRM and press Authenticate Now.
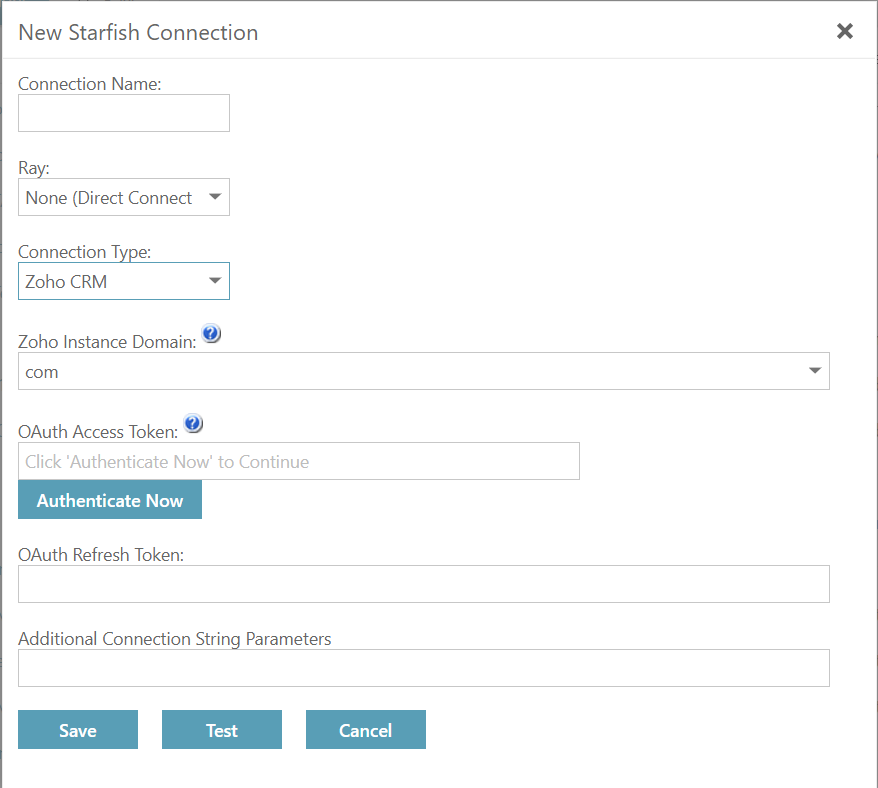
SELECT Statements
A SELECT statement can consist of the following basic clauses.
- SELECT
- INTO
- FROM
- JOIN
- WHERE
- GROUP BY
- HAVING
- UNION
- ORDER BY
- LIMIT
Examples
- Return all columns:
- Rename a column:
SELECT [AccountNumber] AS MY_AccountNumber FROM Accounts
|
- Cast a column's data as a different data type:
SELECT CAST(AnnualRevenue AS VARCHAR) AS Str_AnnualRevenue FROM Accounts
|
- Search data:
SELECT * FROM Accounts WHERE Industry = 'Data/Telecom OEM' ;
|
- Return the number of items matching the query criteria:
SELECT COUNT(*) AS MyCount FROM Accounts
|
- Return the number of unique items matching the query criteria:
SELECT COUNT(DISTINCT AccountNumber) FROM Accounts
|
- Return the unique items matching the query criteria:
SELECT DISTINCT AccountNumber FROM Accounts
|
- Summarize data:
SELECT AccountNumber, MAX(AnnualRevenue) FROM Accounts GROUP BY AccountNumber
|
See Aggregate Functions for details. - Retrieve data from multiple tables.
SELECT Accounts.AccountName, Contacts.FirstName, Contacts.LastName FROM Accounts, Contacts WHERE Accounts.AccountId=Contacts.AccountId
|
See JOIN Queries for details. - Sort a result set in ascending order:
SELECT AccountName, AccountNumber FROM Accounts ORDER BY AccountNumber ASC
|
- Restrict a result set to the specified number of rows:
SELECT AccountName, AccountNumber FROM Accounts LIMIT 10
|
- Parameterize a query to pass in inputs at execution time. This enables you to create prepared statements and mitigate SQL injection attacks.
SELECT * FROM Accounts WHERE Industry = @param
|